What did you do?!?!?
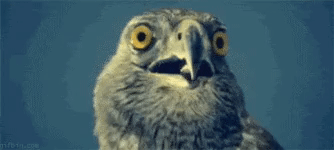
Error: = $errorMsg ?>
'Hacking', 2 => 'Chat Abuse', 3 => 'Gameplay' ); /** PARSE DB CONNECTIONS */ $dbConfigFile = new SplFileObject('database-config.dat'); if ($dbConfigFile->isFile()) { while (!$dbConfigFile->eof()) { $line = trim($dbConfigFile->fgets()); if ($line) // check not empty line { $parts = explode(' ', $line); $fullUrl = $parts[1]; $urlParts = explode('/', $fullUrl); $host = $urlParts[0]; $port = 3306; // check is port has been declared if (strpos($host, ':') !== false) { $hostParts = explode(':', $host); $host = $hostParts[0]; $port = $hostParts[1]; } $database = $urlParts[1]; $name = $parts[0]; $username = $parts[2]; $password = $parts[3]; $connection = new mysqli($host, $username, $password, $database, $port); if ($connection->connect_error) { die("Connection \"$name\" failed: $connection->connect_error"); } $connections[$name] = $connection; } } } else { die('database-config.dat does not exist or is not a file.'); } /** * @param String $name * @return mysqli */ function getConnection($name) { global $connections; return $connections[$name]; } /** * @param Int $reportId * @return Report */ function getReport($reportId) { $connection = getConnection("ACCOUNT"); $statement = $connection->prepare('SELECT reports.suspectId, reports.categoryId, reportHandlers.handlerId FROM reports LEFT JOIN reportHandlers ON reports.id = reportHandlers.reportId AND reportHandlers.aborted IS FALSE LEFT JOIN reportResults ON reports.id = reportResults.reportId WHERE reports.id = ?;'); $statement->bind_param('i', $reportId); $statement->execute(); $statement->store_result(); $statement->bind_result($suspectId, $categoryId, $handlerId); if ($statement->fetch()) { $suspectUser = getUser($suspectId); $reportReasons = getReporters($reportId); $handlerUser = null; if (!is_null($handlerId)) { $handlerUser = getUser($handlerId); } return new Report($reportId, $handlerUser, $suspectUser, $reportReasons, $categoryId); } $statement->close(); return null; } /** * @param string $token * @return int|null */ function getSnapshotId($token) { $connection = getConnection('ACCOUNT'); $statement = $connection->prepare('SELECT id FROM snapshots WHERE token = ?;'); $statement->bind_param('s', $token); $statement->execute(); $statement->bind_result($snapshotId); $statement->store_result(); $statement->fetch(); return $snapshotId; } /** * @param int $snapshotId * @return int|null */ function getSnapshotReportId($snapshotId) { $connection = getConnection('ACCOUNT'); $statement = $connection->prepare('SELECT id FROM reports WHERE snapshotId = ?;'); $statement->bind_param('i', $snapshotId); $statement->execute(); $statement->bind_result($reportId); $statement->store_result(); $statement->fetch(); return $reportId; } function getSnapshot($snapshotId) { /** @var $messages Message[] */ $messages = array(); $connection = getConnection("ACCOUNT"); $statement = $connection->prepare("SELECT messageId, senderId, snapshotType, `server`, `time`, message FROM snapshots, snapshotMessages, snapshotMessageMap WHERE snapshotMessageMap.snapshotId = snapshots.id AND snapshotMessages.id = snapshotMessageMap.messageId AND snapshots.id = ?;"); $statement->bind_param('i', $snapshotId); $statement->execute(); $statement->bind_result($snapshotId, $senderId, $snapshotType, $server, $time, $message); $statement->store_result(); while ($statement->fetch()) { $recipients = getUsers(getMessageRecipients($snapshotId)); $message = new Message(getUser($senderId), $recipients, $time, $snapshotType, $message, $server); array_push($messages, $message); } $statement->close(); $snapshotUsers = array(); foreach ($messages as $message) { $sender = $message->getSender(); $snapshotUsers[$sender->getId()] = $sender; foreach ($message->getRecipients() as $recipient) { $snapshotUsers[$recipient->getId()] = $recipient; } } return new Snapshot($snapshotId, $messages, $snapshotUsers); } /** * @param $snapshotId * @return Integer[] array */ function getMessageRecipients($snapshotId) { $recipientIds = array(); $connection = getConnection("ACCOUNT"); $statement = $connection->prepare("SELECT recipientId FROM snapshotRecipients WHERE messageId = ?"); $statement->bind_param('i', $snapshotId); $statement->execute(); $statement->bind_result($recipientId); while ($statement->fetch()) { array_push($recipientIds, $recipientId); } $statement->close(); return $recipientIds; } /** * @param Integer[] $ids * @return User[] array */ function getUsers($ids) { $users = array(); foreach ($ids as $id) { array_push($users, getUser($id)); } return $users; } /** * @param $id * @return User */ function getUser($id) { if (isset($users[$id])) { return $users[$id]; } else { $connection = getConnection("ACCOUNT"); $statement = $connection->prepare('SELECT uuid, `name`, rank FROM accounts WHERE id = ?'); $statement->bind_param('i', $id); $statement->execute(); $statement->bind_result($uuid, $name, $rank); $statement->fetch(); $user = new User($id, $uuid, $name, parseRank($rank)); $users[$id] = $user; $statement->close(); return $user; } } /** * @param int $reportId * @return UserReport[] */ function getReporters($reportId) { global $dateTimeZone; $connection = getConnection("ACCOUNT"); $statement = $connection->prepare("SELECT reporterId, `time`, reason FROM reportReasons WHERE reportId = ?"); $reportReasons = array(); $statement->bind_param('i', $reportId); $statement->execute(); $statement->bind_result($reporterId, $time, $reason); $statement->store_result(); // prevents issues with other queries running before this statement is closed while ($statement->fetch()) { $reportReasons[$reporterId] = new UserReport(getUser($reporterId), new DateTime($time, $dateTimeZone), $reason); } $statement->close(); return $reportReasons; } /** * @param Report $report * @return User[] */ function getInvolvedUsers($report) { $involvedUsers[$report->getSuspect()->getId()] = $report->getSuspect(); foreach ($report->getReporters() as $reporterReason) { $reporter = $reporterReason->getUser(); $involvedUsers[$reporter->getId()] = $reporter; } return $involvedUsers; } /** * @param string $dbRank * @return string */ function parseRank($dbRank) { $rank = $dbRank; if ($dbRank == 'ALL') { $rank = 'PLAYER'; } return $rank; } /** * @param Message $messageA * @param Message $messageB * @return int */ function compareMessageTimes($messageA, $messageB) { return $messageA->getTimestamp()->getTimestamp() - $messageB->getTimestamp()->getTimestamp(); } /** * @param String $dateTime * @param DateTimeZone $timezone * @return DateTime */ function parseDateTime($dateTime, $timezone) { return DateTime::createFromFormat(jsonDateTimeFormat, $dateTime, $timezone); } /** * Converts an interval to minutes, days or months, depending on the size. * * @param DateInterval $interval * @return string */ function approximateHumanInterval($interval) { if ($interval->y > 0) { $humanString = $interval->y . ' year' . ($interval->y != 1 ? 's' : ''); } else if ($interval->m > 0) { $humanString = $interval->m . ' month' . ($interval->m != 1 ? 's' : ''); } else if ($interval->d > 0) { $humanString = $interval->d . ' day' . ($interval->d != 1 ? 's' : ''); } else if ($interval->h > 0) { $humanString = $interval->h . ' hour' . ($interval->h != 1 ? 's' : ''); } else if ($interval->i > 0) { $humanString = $interval->i . ' minute' . ($interval->i != 1 ? 's' : ''); } else { $humanString = $interval->s . ' second' . ($interval->s != 1 ? 's' : ''); } return $humanString; } function getExpandedURL() { $vars = $_GET; $vars['expanded'] = true; return '?' . http_build_query($vars); } $validToken = isset($_GET['token']); $errorMsg = ""; $title = 'Report & Snapshot System'; $token = null; $expanded = null; $report = null; $snapshot = null; $messages = null; if ($validToken) { $token = $_GET['token']; $expanded = isset($_GET['expanded']) && $_GET['expanded']; $snapshotId = getSnapshotId($token); if ($snapshotId != null) { $snapshot = getSnapshot($snapshotId); $messages = $snapshot->getMessages(); $reportId = getSnapshotReportId($snapshotId); $report = null; if ($reportId) { $report = getReport($reportId); $title = "Report #$reportId"; } else { $title = "Snapshot #$snapshotId"; } } else { $validToken = false; $errorMsg = 'Invalid token.'; } } ?>
What did you do?!?!?
Error: = $errorMsg ?>
= $user->getUUID() ?>